Stripe Tokenization Flow
Tokenize the credit card in Stripe to use it for guest payment.
Overview
There are three primary steps to creating a guest payment method for reservations.
- Determine the integrated payment processor account that is assigned to the listing.
- Collect and tokenize the guest's credit card in the Stripe account identified in the first step.
- Add it as the
ccToken
in the reservation creation request.
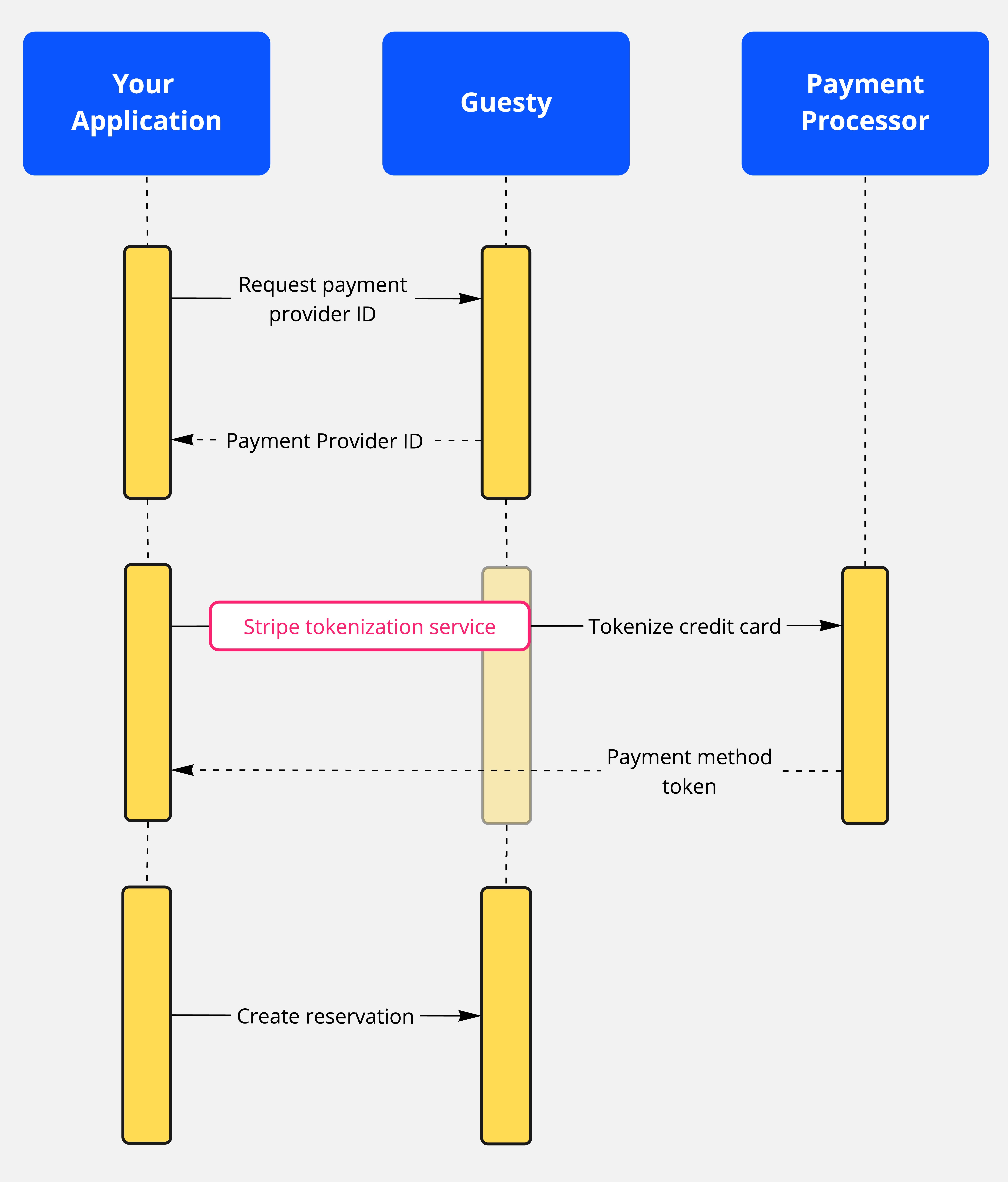
Figure 1.0. Create a Guest Payment Method Flow
Connect a Stripe Account
Before Guesty can process Stripe payments for bookings, a Stripe account must be connected to your Guesty account. Learn more.
Step 1: Identify the Payment Processor Account
The payment method token must come from the same payment processor account integrated with your Guesty account and assigned to the relevant listing. Use the following request to retrieve the details of the Stripe account assigned to the property:
Example
Request
curl --globoff 'https://booking.guesty.com/api//listings/{id}/payment-provider' \
--header 'accept: application/json; charset=utf-8' \
--header 'Authorization: Bearer {token}'
const myHeaders = new Headers();
myHeaders.append("accept", "application/json; charset=utf-8");
myHeaders.append("Authorization", "Bearer {token}");
const requestOptions = {
method: "GET",
headers: myHeaders,
redirect: "manual"
};
fetch("https://booking.guesty.com/api//listings/{id}/payment-provider", requestOptions)
.then((response) => response.text())
.then((result) => console.log(result))
.catch((error) => console.error(error));
var https = require('follow-redirects').https;
var fs = require('fs');
var options = {
'method': 'GET',
'hostname': 'booking.guesty.com',
'path': '/api//listings/{id}/payment-provider',
'headers': {
'accept': 'application/json; charset=utf-8',
'Authorization': 'Bearer {token}'
},
'maxRedirects': 20
};
var req = https.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function (chunk) {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
res.on("error", function (error) {
console.error(error);
});
});
req.end();
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://booking.guesty.com/api//listings/{id}/payment-provider',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => false,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'accept: application/json; charset=utf-8',
'Authorization: Bearer {token}'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
import http.client
conn = http.client.HTTPSConnection("booking.guesty.com")
payload = ''
headers = {
'accept': 'application/json; charset=utf-8',
'Authorization': 'Bearer {token}'
}
conn.request("GET", "/api//listings/{id}/payment-provider", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
Response
{
"_id": "string",
"providerType": "stripe",
"providerAccountId": "acct_<string>",
"status": "ACTIVE",
"paymentProcessorName": "Stripe",
"accountName": "Main Stripe Account",
"paymentProcessorId": "string"
}
Step 2: Tokenize the Guest's Card
Generate a payment method token from the connected Stripe account assigned to the Guesty listing. You will need to develop your own integration with your application for this purpose.
Note
Take care to create only the payment token in Stripe, and not the customer (guest). Guesty will create the guest the first time it charges the card.
Step 3: Create the Reservation
Pass the Stripe payment token as the ccToken
in the inquiry or instant booking request.
Reuse of Tokens
Using the same payment token for multiple reservations is not supported. It will result in a missing payment method error in the user dashboard (even though it exists), preventing auto payments from being executed as scheduled. Generate a new token for each new inquiry or reservation through the Booking Engine API.
Troubleshooting
The reservation was created without a payment method
This may be due to either of the following:
- The credit card was tokenized in a different payment processor account than the one assigned to the listing. Determine the correct account using the Get payment provider by listing and Get provider stats.
- The payment processor or credit card issuer reported an issue. Check your payment processor extranet for error messages and see our Help Center troubleshooting articles.
How can I be sure the payment method succeeded?
Retrieve the reservation object. Its payload will show the payment method object nested under the money.payments
section.
Stripe Payment Error: This PaymentMethod was previously used without being attached to a Customer or was detached from a Customer and may not be used again.
This occurred because you created the customer in Stripe. This links the token with that customer and prevents Guesty from using it. You must only create the payment token in Stripe. Guesty will create the Stripe customer when it validates the token.
You can prevent this by implementing the following:
- Omit the pre-charge and subscribe to the payment.failed webhook to monitor for failed payments on the reservations.
- Tokenize the card again after you have completed the check and send Guesty a fresh pm token
Updated 8 months ago